ZOO-Project
|
response_print.h
Go to the documentation of this file.
83 #include <locale.h> // knut: this appears to be a non-standard header file that has been removed in newer versions of glibc; it may be sufficient to include <locale.h> (see above)
148 {"1.0.0","http://www.opengis.net/ows/1.1","http://www.opengis.net/wps/1.0.0","http://schemas.opengis.net/wps/1.0.0","%s %s/wps%s_response.xsd","http://schemas.opengis.net/ows/1.1.0/owsExceptionReport.xsd","1.1.0"},
149 {"2.0.0","http://www.opengis.net/ows/2.0","http://www.opengis.net/wps/2.0","http://schemas.opengis.net/wps/2.0","http://www.opengis.net/wps/2.0 http://schemas.opengis.net/wps/2.0/wps.xsd","http://schemas.opengis.net/ows/2.0/owsExceptionReport.xsd","2.0.2","http://www.opengis.net/spec/wps/2.0/def/process-profile/"},
248 void printFullDescription(xmlDocPtr,int,elements*,const char*,xmlNsPtr,xmlNsPtr,xmlNodePtr,int,int,const map*);
251 void printIOType(xmlDocPtr,xmlNodePtr,xmlNsPtr,xmlNsPtr,xmlNsPtr,elements*,maps*,const char*,int);
256 void printOutputDefinitions(xmlDocPtr,xmlNodePtr,xmlNsPtr,xmlNsPtr,elements*,maps*,const char*);
xmlNodePtr printWPSHeader(xmlDocPtr, maps *, const char *, const char *, const char *, int)
Generate a WPS header.
Definition: response_print.c:343
void zooXmlCleanupDocs()
Free allocated memort to store XML documents.
Definition: response_print.c:292
static const char * schemas[2][8]
Definitions of schemas depending on the WPS version.
Definition: response_print.h:147
int zooXmlSearchForNs(const char *)
Search for an existing XML namespace in usedNS.
Definition: response_print.c:214
static xmlDocPtr iDocs[ZOO_DOC_MAX]
Array of xmlDocPtr storing XML docs.
Definition: response_print.h:130
void printIOType(xmlDocPtr, xmlNodePtr, xmlNsPtr, xmlNsPtr, xmlNsPtr, elements *, maps *, const char *, int)
Generate XML nodes describing inputs or outputs metadata.
Definition: response_print.c:2262
map * parseBoundingBox(const char *)
Parse a BoundingBox string.
Definition: response_print.c:3134
void outputResponse(service *, maps *, maps *, map *, int, maps *, int)
Generate the output response (RawDataOutput or ResponseDocument)
Definition: response_print.c:2850
int errorException(maps *, const char *, const char *, const char *)
Print an OWS ExceptionReport.
Definition: response_print.c:2720
void printDescribeProcessForProcess(registry *, maps *, void *, void *, service *)
Generate a ProcessDescription node for a servie and add it to a given node.
Definition: response_print.c:1069
const char * produceStatusString(maps *, map *)
Produce the status string used in HTTP headers.
Definition: response_print.c:2542
static xmlNsPtr usedNs[ZOO_NS_MAX]
Array of xmlNsPtr storing all used XML namespace.
Definition: response_print.h:118
void zooXmlCleanupNs()
Free allocated memory to store used XML namespace.
Definition: response_print.c:258
static int nbSupportedRequests
Definitions of support requests (depending on the WPS version)
Definition: response_print.h:154
void printGetCapabilitiesForProcess(registry *, maps *, void *, void *, service *)
Generate a wps:Process node for a servie and add it to a given node.
Definition: response_print.c:706
void addLangAttr(xmlNodePtr, maps *)
Add a land attribute to a XML node.
Definition: response_print.c:170
void addAdditionalParameters(map *, xmlDocPtr, xmlNodePtr, xmlNsPtr, xmlNsPtr, int)
Add AdditionalParameters nodes to any existing node.
Definition: response_print.c:911
void printFullDescription(xmlDocPtr, int, elements *, const char *, xmlNsPtr, xmlNsPtr, xmlNodePtr, int, int, const map *)
Generate the required XML tree for the detailled metadata information of inputs or outputs...
Definition: response_print.c:1188
int checkForSoapEnvelope(xmlDocPtr)
int zooXmlAddNs(xmlNodePtr, const char *, const char *)
Add an XML namespace to the usedNS if it was not already used.
Definition: response_print.c:233
static const char * requests[2][7]
Definitions of requests depending on the WPS version.
Definition: response_print.h:158
void printBoundingBoxDocument(maps *, maps *, FILE *)
Print an ows:BoundingBox XML document.
Definition: response_print.c:3187
static const char * capabilities[2][7]
Name and corresponding attributes depending on the WPS version.
Definition: response_print.h:181
void printOutputDefinitions(xmlDocPtr, xmlNodePtr, xmlNsPtr, xmlNsPtr, elements *, maps *, const char *)
Print a XML document.
Definition: response_print.c:2218
void printHeaders(maps *)
Print the HTTP headers based on a map.
Definition: response_print.c:89
void addMetadata(map *, xmlDocPtr, xmlNodePtr, xmlNsPtr, xmlNsPtr, int)
Add a Metadata node to any existing node.
Definition: response_print.c:824
static int nbReqJob
Definitions requests requiring jobid (only for WPS version 2.0.0)
Definition: response_print.h:169
static char wpsStatus[3][11]
Definitions of acceptable final status.
Definition: response_print.h:139
void printBoundingBox(xmlNsPtr, xmlNodePtr, map *)
Create required XML nodes for boundingbox and update the current XML node.
Definition: response_print.c:3082
void printDescription(xmlNodePtr, xmlNsPtr, const char *, map *, int)
Create XML node with basic ows metadata information (Identifier,Title,Abstract)
Definition: response_print.c:2509
static char * SERVICE_URL
Global char* to store the serverAddress value of the [main] section.
Definition: response_print.h:113
char * produceFileUrl(service *, maps *, maps *, const char *, int)
Produce a copy file and the corresponding url in case it is required Please, free the returned ressou...
Definition: response_print.c:2746
void printProcessResponse(maps *, map *, int, service *, const char *, int, maps *, maps *)
Generate a wps:Execute XML document.
Definition: response_print.c:1865
xmlNodePtr soapEnvelope(maps *, xmlNodePtr)
Generate a SOAP Envelope node when required (if the isSoap key of the [main] section is set to true)...
Definition: response_print.c:309
void printDocument(maps *, xmlDocPtr, int)
Print a XML document.
Definition: response_print.c:2183
void printExceptionReportResponse(maps *, map *)
Print an OWS ExceptionReport or exception.yaml Document and HTTP headers (when required) depending on...
Definition: response_print.c:2622
void printSessionHeaders(maps *)
Print the Set-Cookie header if necessary (conf["lenv"]["cookie"]) and save the session file...
Definition: response_print.c:109
xmlNodePtr printGetCapabilitiesHeader(xmlDocPtr, maps *, const char *)
Generate a Capabilities header.
Definition: response_print.c:441
xmlNodePtr createExceptionReportNode(maps *, map *, int)
Create an OWS ExceptionReport Node.
Definition: response_print.c:2641
static int nbReqIdentifier
Definitions requests requiring identifier (depending on the WPS version)
Definition: response_print.h:165
static char * nsName[ZOO_NS_MAX]
Array storing names of the used XML namespace.
Definition: response_print.h:122
void printStatusInfo(maps *, map *, char *)
Print a StatusInfo XML document.
Definition: response_print.c:3251
int zooXmlAddDoc(xmlNodePtr, const char *, const char *)
static const char * root_nodes[2][4]
Definitions of root node for response depending on the request and the WPS version.
Definition: response_print.h:173
void * printRawdataOutput(maps *, maps *)
Print one outputs as raw.
Definition: response_print.c:3025
Generated on Wed Feb 5 2025 13:01:44 for ZOO-Project by
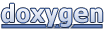