ZOO-Project
|
#include "request_parser.h"
#include "service_internal.h"
#include "server_internal.h"
#include "response_print.h"
#include "caching.h"
#include "cgic.h"
Functions | |
xmlXPathObjectPtr | extractFromDoc (xmlDocPtr doc, const char *search) |
Apply XPath Expression on XML document. More... | |
int | appendMapsToMaps (maps *m, maps *mo, maps *mi, elements *elem) |
Create (or append to) an array valued maps value = "["",""]". More... | |
void | ensureDecodedBase64 (maps **in) |
Make sure that each value encoded in base64 in a maps is decoded. More... | |
int | kvpParseInputs (maps **main_conf, service *s, map *request_inputs, maps **request_output, HINTERNET *hInternet) |
Parse inputs provided as KVP and store them in a maps. More... | |
int | kvpParseOutputs (maps **main_conf, map *request_inputs, maps **request_output) |
Parse outputs provided as KVP and store them in a maps. More... | |
int | defineMissingIdentifier (maps **main_conf, maps **mymaps) |
Create a "missingIdentifier" maps in case it is NULL. More... | |
int | xmlParseInputs (maps **main_conf, service *s, maps **request_output, xmlDocPtr doc, xmlNodeSet *nodes, HINTERNET *hInternet) |
Parse inputs from XML nodes and store them in a maps. More... | |
int | xmlParseBoundingBox (maps **main_conf, map **current_input, xmlDocPtr doc) |
Parse a BoundingBoxData node. More... | |
int | xmlParseOutputs2 (maps **main_conf, map **request_inputs, maps **request_output, xmlDocPtr doc, xmlNodeSet *nodes) |
Parse outputs from XML nodes and store them in a maps (WPS version 2.0.0). More... | |
int | xmlParseOutputs (maps **main_conf, map **request_inputs, maps **request_output, xmlDocPtr doc, xmlNodePtr cur, bool raw) |
Parse outputs from XML nodes and store them in a maps. More... | |
int | xmlParseRequest (maps **main_conf, const char *post, map **request_inputs, service *s, maps **inputs, maps **outputs, HINTERNET *hInternet) |
Parse XML request and store information in maps. More... | |
int | parseRequest (maps **main_conf, map **request_inputs, service *s, maps **inputs, maps **outputs, HINTERNET *hInternet) |
Parse request and store information in maps. More... | |
int | validateRequest (maps **main_conf, service *s, map *original_request, maps **request_inputs, maps **request_outputs, HINTERNET *hInternet) |
Ensure that each requested arguments are present in the request DataInputs and ResponseDocument / RawDataOutput. More... | |
void | checkValidValue (map *request, map **res, const char *toCheck, const char **avalues, int mandatory) |
Verify if a parameter value is valid. More... | |
void | parseCookie (maps **conf, const char *cookie) |
Parse cookie contained in request headers. More... | |
Function Documentation
Create (or append to) an array valued maps value = "["",""]".
- Parameters
-
m the conf maps containing the main.cfg settings mo the map to update mi the map to append elem the elements containing default definitions
- Returns
- 0 on success, -1 on failure
void checkValidValue | ( | map * | request, |
map ** | res, | ||
const char * | toCheck, | ||
const char ** | avalues, | ||
int | mandatory | ||
) |
Verify if a parameter value is valid.
- Parameters
-
request the request map res the error map potentially generated toCheck the parameter to use avalues the acceptable values (or null if testing only for presence) mandatory verify the presence of the parameter if mandatory > 0
Create a "missingIdentifier" maps in case it is NULL.
- Parameters
-
main_conf the conf maps containing the main.cfg settings mymaps the maps to update
- Returns
- 0 on success, 4 on failure
void ensureDecodedBase64 | ( | maps ** | in | ) |
Make sure that each value encoded in base64 in a maps is decoded.
- Parameters
-
in the maps containing the values
- See also
- readBase64
xmlXPathObjectPtr extractFromDoc | ( | xmlDocPtr | doc, |
const char * | search | ||
) |
Apply XPath Expression on XML document.
- Parameters
-
doc the XML Document search the XPath expression
- Returns
- xmlXPathObjectPtr containing the resulting nodes set
int kvpParseInputs | ( | maps ** | main_conf, |
service * | s, | ||
map * | request_inputs, | ||
maps ** | request_output, | ||
HINTERNET * | hInternet | ||
) |
Parse inputs provided as KVP and store them in a maps.
- Parameters
-
main_conf the conf maps containing the main.cfg settings s the service request_inputs the map storing KVP raw value request_output the maps to store the KVP pairs hInternet the HINTERNET queue to add potential requests
- Returns
- 0 on success, -1 on failure
Parse outputs provided as KVP and store them in a maps.
- Parameters
-
main_conf the conf maps containing the main.cfg settings request_inputs the map storing KVP raw value request_output the maps to store the KVP pairs
- Returns
- 0 on success, -1 on failure
Parsing outputs provided as KVP
Put each Output into the outputs_as_text array
void parseCookie | ( | maps ** | conf, |
const char * | cookie | ||
) |
Parse cookie contained in request headers.
- Parameters
-
conf the conf maps containinfg the main.cfg cookie the
int parseRequest | ( | maps ** | main_conf, |
map ** | request_inputs, | ||
service * | s, | ||
maps ** | inputs, | ||
maps ** | outputs, | ||
HINTERNET * | hInternet | ||
) |
Parse request and store information in maps.
- Parameters
-
main_conf the conf maps containing the main.cfg settings post the string containing the XML request request_inputs the map storing KVP raw value s the service inputs the maps to store the KVP pairs outputs the maps to store the KVP pairs hInternet the HINTERNET queue to add potential requests
- Returns
- 0 on success, -1 on failure
int validateRequest | ( | maps ** | main_conf, |
service * | s, | ||
map * | original_request, | ||
maps ** | request_inputs, | ||
maps ** | request_outputs, | ||
HINTERNET * | hInternet | ||
) |
Ensure that each requested arguments are present in the request DataInputs and ResponseDocument / RawDataOutput.
Potentially run http requests from the queue in parallel. For optional inputs add default values defined in the ZCFG file.
- Parameters
-
main_conf s original_request request_inputs request_outputs hInternet
- See also
- runHttpRequests
Parse a BoundingBoxData node.
http://schemas.opengis.net/ows/1.1.0/owsCommon.xsd: BoundingBoxType
A map to store boundingbox information will contain:
- LowerCorner : double double (minimum within this bounding box)
- UpperCorner : double double (maximum within this bounding box)
- crs : URI (Reference to definition of the CRS)
- dimensions : int
- Parameters
-
main_conf the conf maps containing the main.cfg settings request_inputs the map storing KVP raw value doc the xmlDocPtr containing the BoudingoxData node
- Returns
- a map containing all the bounding box keys
int xmlParseInputs | ( | maps ** | main_conf, |
service * | s, | ||
maps ** | request_output, | ||
xmlDocPtr | doc, | ||
xmlNodeSet * | nodes, | ||
HINTERNET * | hInternet | ||
) |
Parse inputs from XML nodes and store them in a maps.
- Parameters
-
main_conf the conf maps containing the main.cfg settings s the service request_output the maps to store the KVP pairs doc the xmlDocPtr containing the original request nodes the input nodes array hInternet the HINTERNET queue to add potential requests
- Returns
- 0 on success, -1 on failure
int xmlParseOutputs | ( | maps ** | main_conf, |
map ** | request_inputs, | ||
maps ** | request_output, | ||
xmlDocPtr | doc, | ||
xmlNodePtr | cur, | ||
bool | raw | ||
) |
Parse outputs from XML nodes and store them in a maps.
- Parameters
-
main_conf the conf maps containing the main.cfg settings request_inputs the map storing KVP raw value request_output the maps to store the KVP pairs doc the xmlDocPtr containing the original request cur the xmlNodePtr corresponding to the ResponseDocument or RawDataOutput XML node raw true if the node is RawDataOutput, false in case of ResponseDocument
- Returns
- 0 on success, -1 on failure
int xmlParseOutputs2 | ( | maps ** | main_conf, |
map ** | request_inputs, | ||
maps ** | request_output, | ||
xmlDocPtr | doc, | ||
xmlNodeSet * | nodes | ||
) |
Parse outputs from XML nodes and store them in a maps (WPS version 2.0.0).
- Parameters
-
main_conf the conf maps containing the main.cfg settings request_inputs the map storing KVP raw value request_output the maps to store the KVP pairs doc the xmlDocPtr containing the original request cur the xmlNodePtr corresponding to the ResponseDocument or RawDataOutput XML node raw true if the node is RawDataOutput, false in case of ResponseDocument
- Returns
- 0 on success, -1 on failure
int xmlParseRequest | ( | maps ** | main_conf, |
const char * | post, | ||
map ** | request_inputs, | ||
service * | s, | ||
maps ** | inputs, | ||
maps ** | outputs, | ||
HINTERNET * | hInternet | ||
) |
Parse XML request and store information in maps.
- Parameters
-
main_conf the conf maps containing the main.cfg settings post the string containing the XML request request_inputs the map storing KVP raw value s the service inputs the maps to store the KVP pairs outputs the maps to store the KVP pairs hInternet the HINTERNET queue to add potential requests
- Returns
- 0 on success, -1 on failure
Extract Input nodes from the XML Request.
Generated on Fri Apr 25 2025 05:00:01 for ZOO-Project by
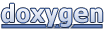